“Coding Druid” series is my horizontal programming practice notes, each part around a topic like mathematical, physics, electronic, graphics, sound, etc., implemented in several programming languages.
Coding Druid
Part: Math
Chapter: Fourier Series
Section: Python (in Blender)
In this Chapter, I have visualized Fourier Series separately using JavaScript (React):
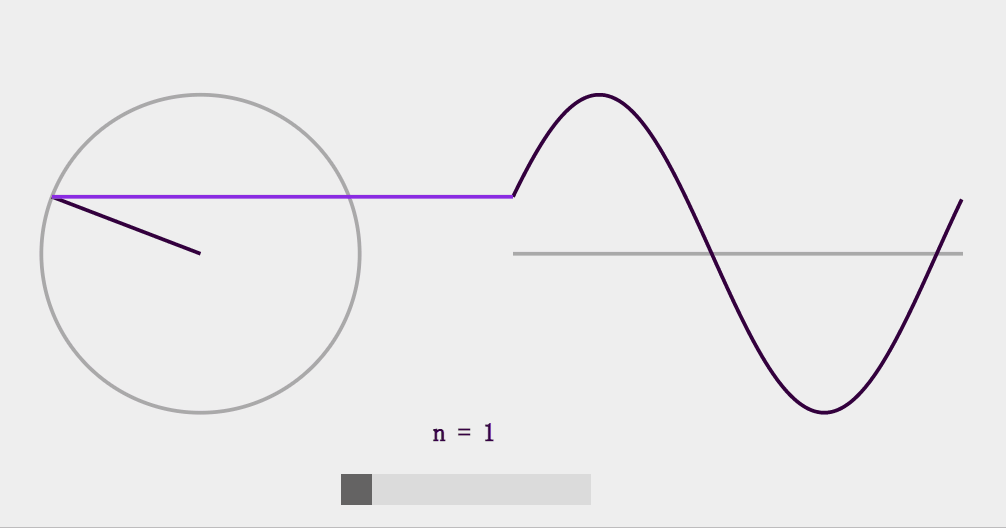
and Unity:
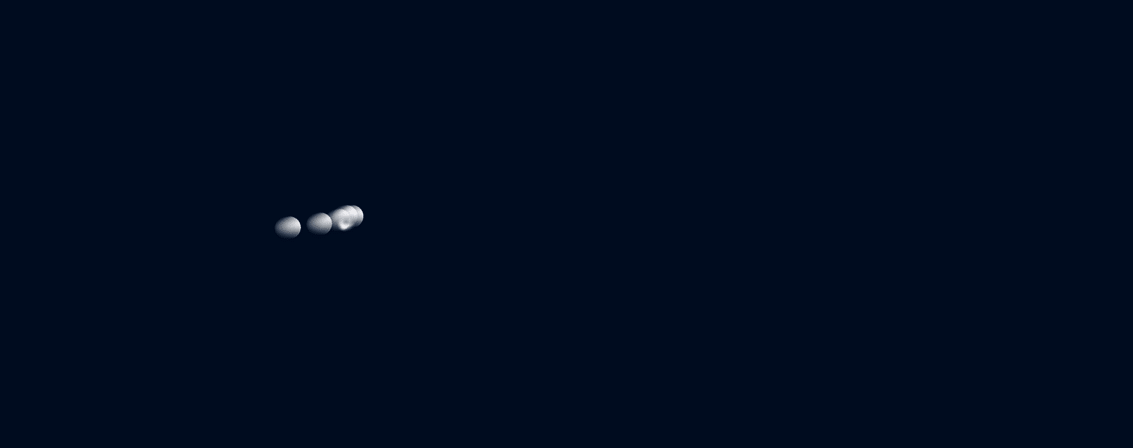
This section is Python again.
Playground options
In the note Sine function visualization using Python I used Jupyter Notebook as Python IDE/Playground.
Jupyter is a great online IDE for Python. You can basically do real-time programming, see the running results as you write, and mix code and documentation.
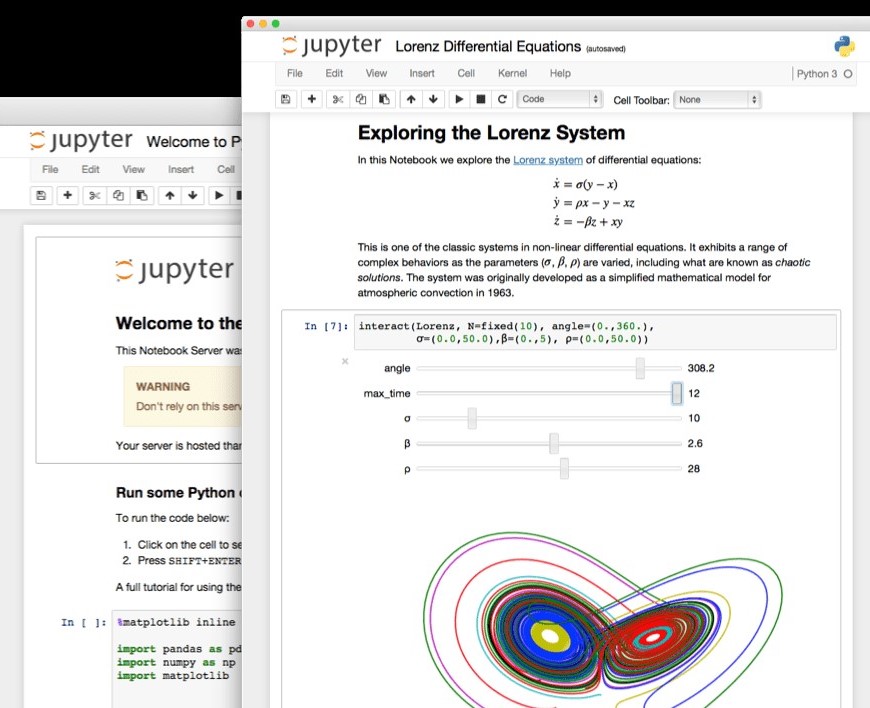
This will be easy, without having to think about how to set up a local Python environment, or how to visualize/render the results, and so on.
Just focus on Python code first, and write and view the results directly in the browser.
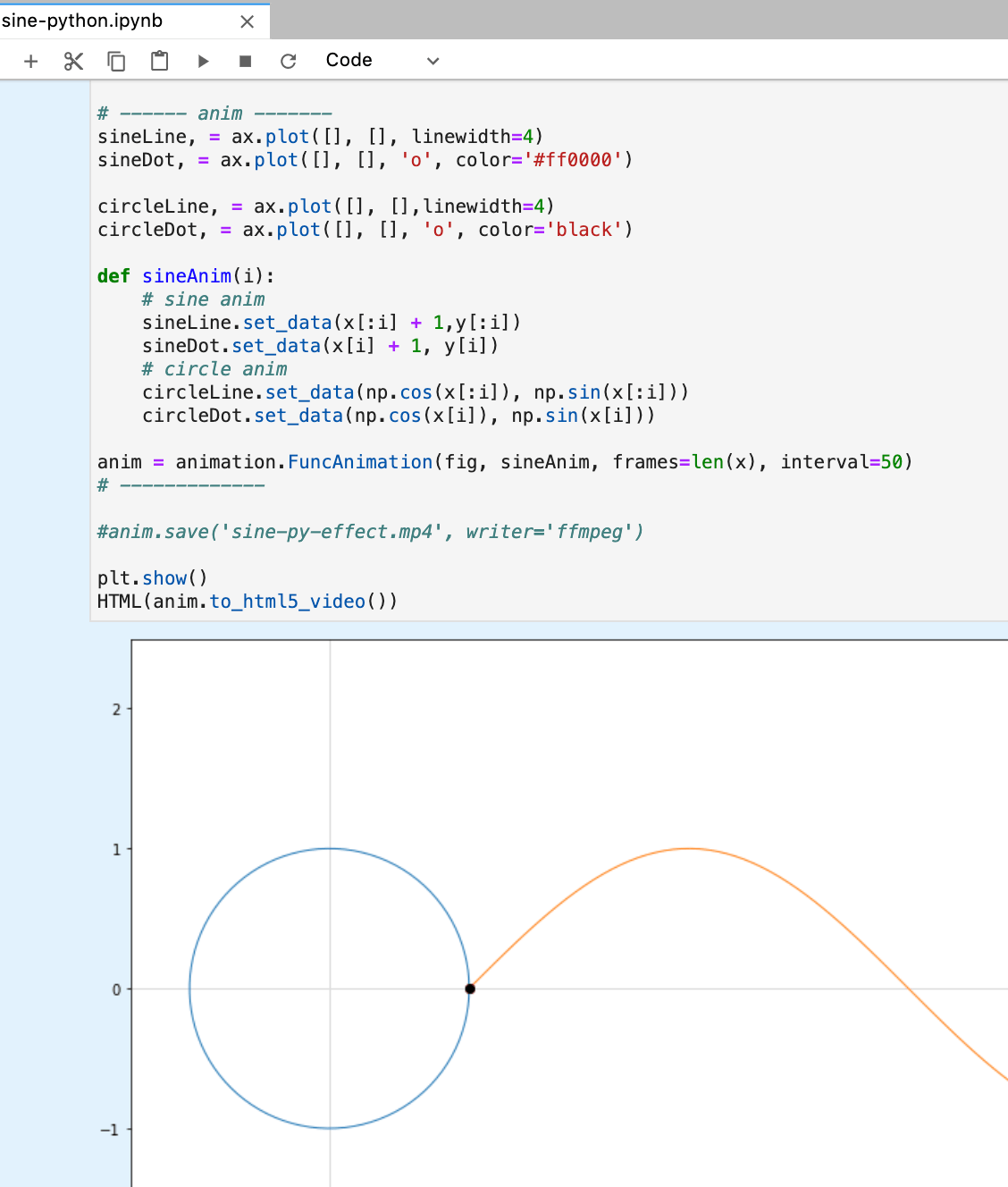
Jupyter is good, but it is not wild enough. If you want to do richer visualizations, instead of just drawing formula curves, then using Jupyter to run Python is a bit limited.
I need a Python runtime that does not have to worry too much about the implementation of graphics rendering. And also need the rendering effect is powerful and rude.
Blender and TouchDesigner are two software that meet this criteria.
Blender
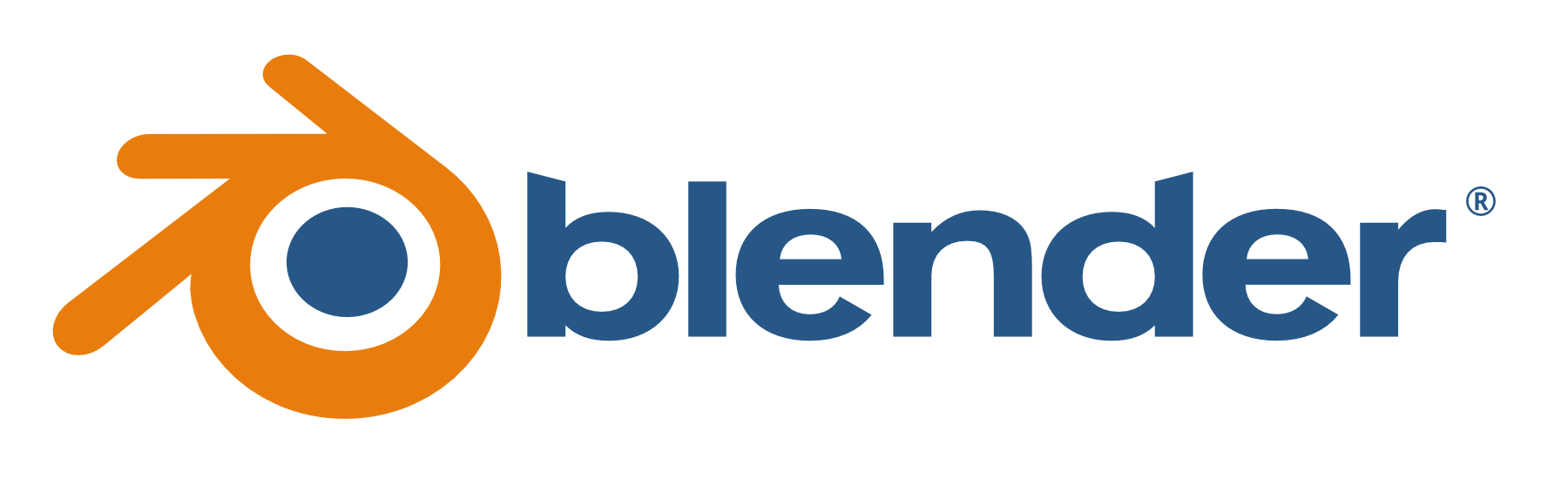
Blender is an open source 3D content creation software. Its core functions can be compared to 3D Max, Maya, etc. It is size-small and simple but has rough functions. It crosses multiple operating systems. For details, see its official website:
https://www.blender.org/
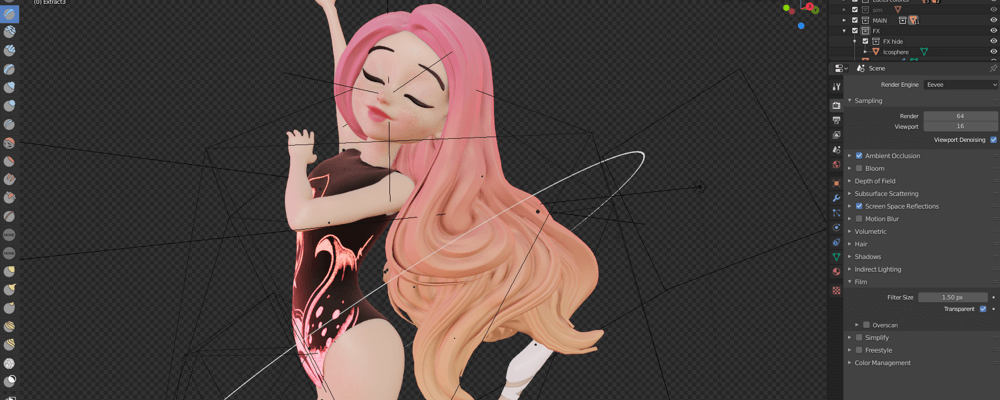
Blender’s ecosystem is built on Python. Plugins, extensions, advanced customization features, and more can be written in Python.
In fact, you can also do real-time interactive games, which is a bit like Unity, of course, Blender is more focused on the production of 3D content.
TouchDesigner
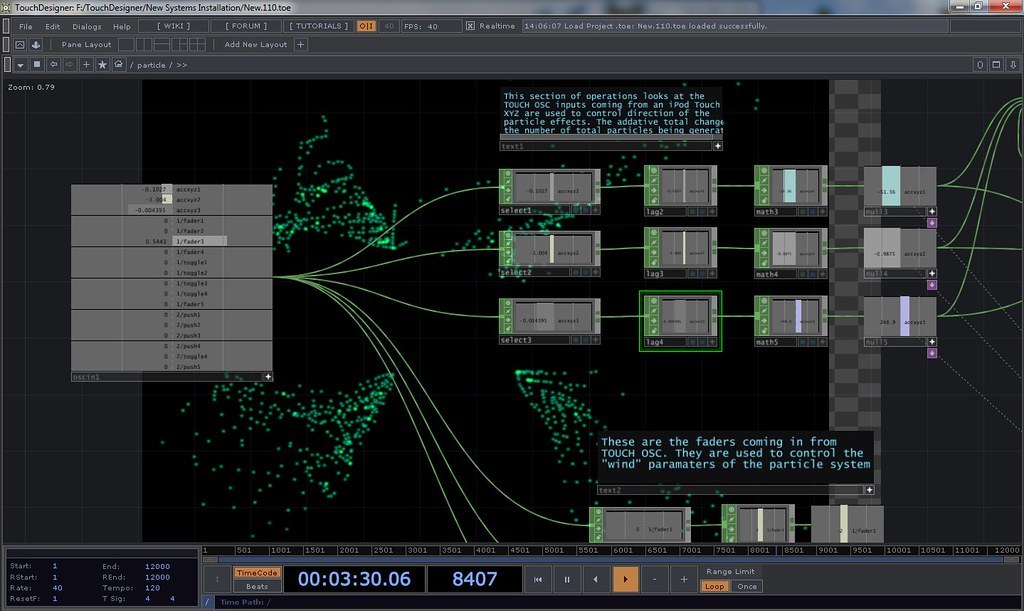
TouchDesigner is a graphical programming tool that can be compared to other tools such as [MaxMSP] (https://cycling74.com/), [vvvv] (https://vvvv.org/), etc.
TouchDesigner’s extended scripting language is also Python.
For me, playing graphical programming with MaxMSP is enough for now.
So this time I use Blender as the IDE for Python programming.
Hello World
Firstly download the 2.8x installation file on Blender’s official website. Mac, Windows, and Linux all are supported.
Blender has a huge update since version 2.80. I don’t know exactly how big it is, because the last time I used Blender more than ten years ago. It was still version 2.4x, and I tried to use Python on Parametric Building Generation.
Anyway, I just use version 2.80 as a new software.
Lauched after installation:
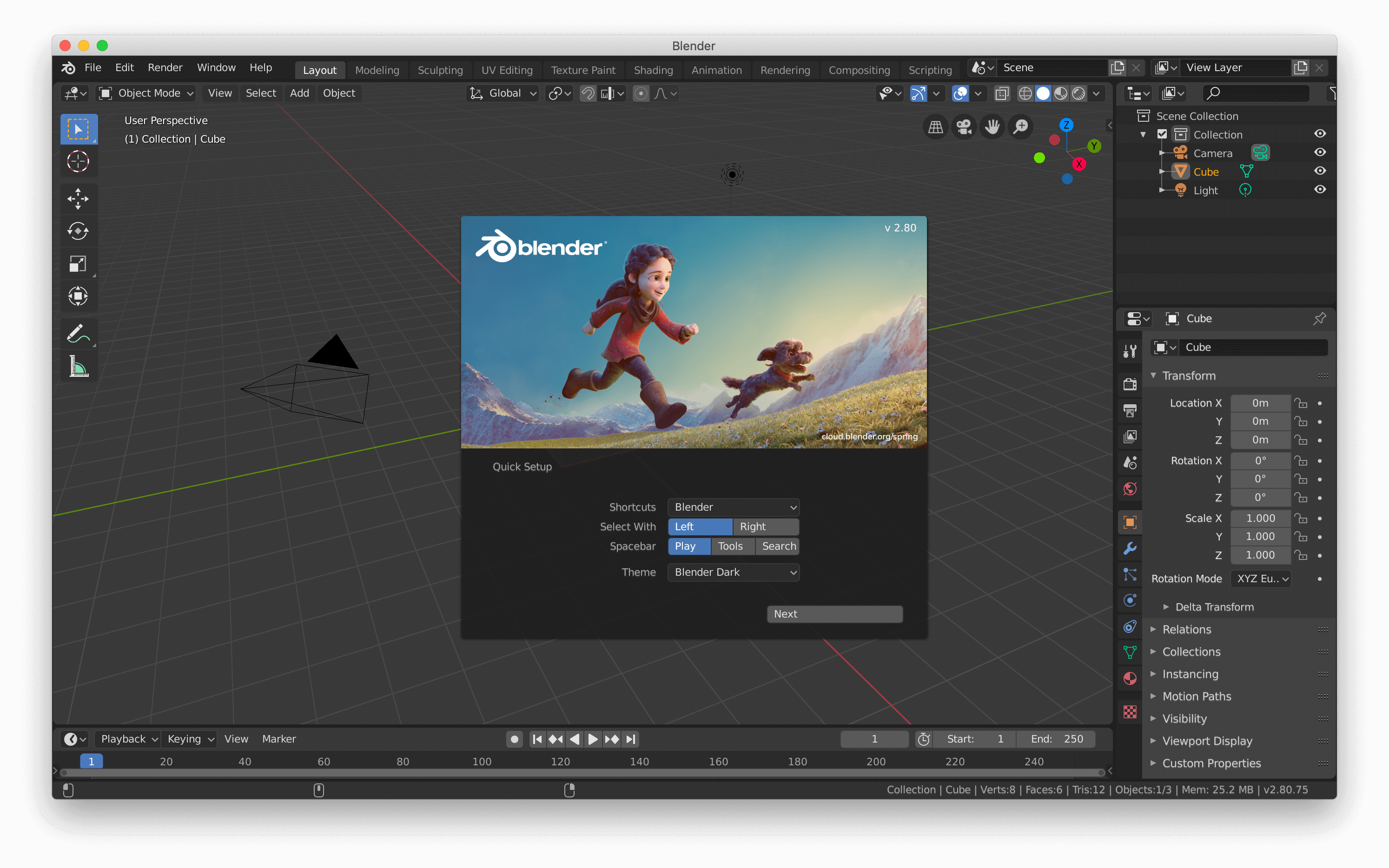
It has many functions, such as modeling, mapping, animation, rendering, etc. It is recommended to check the official tutorial.
This article is just focus on running Python.
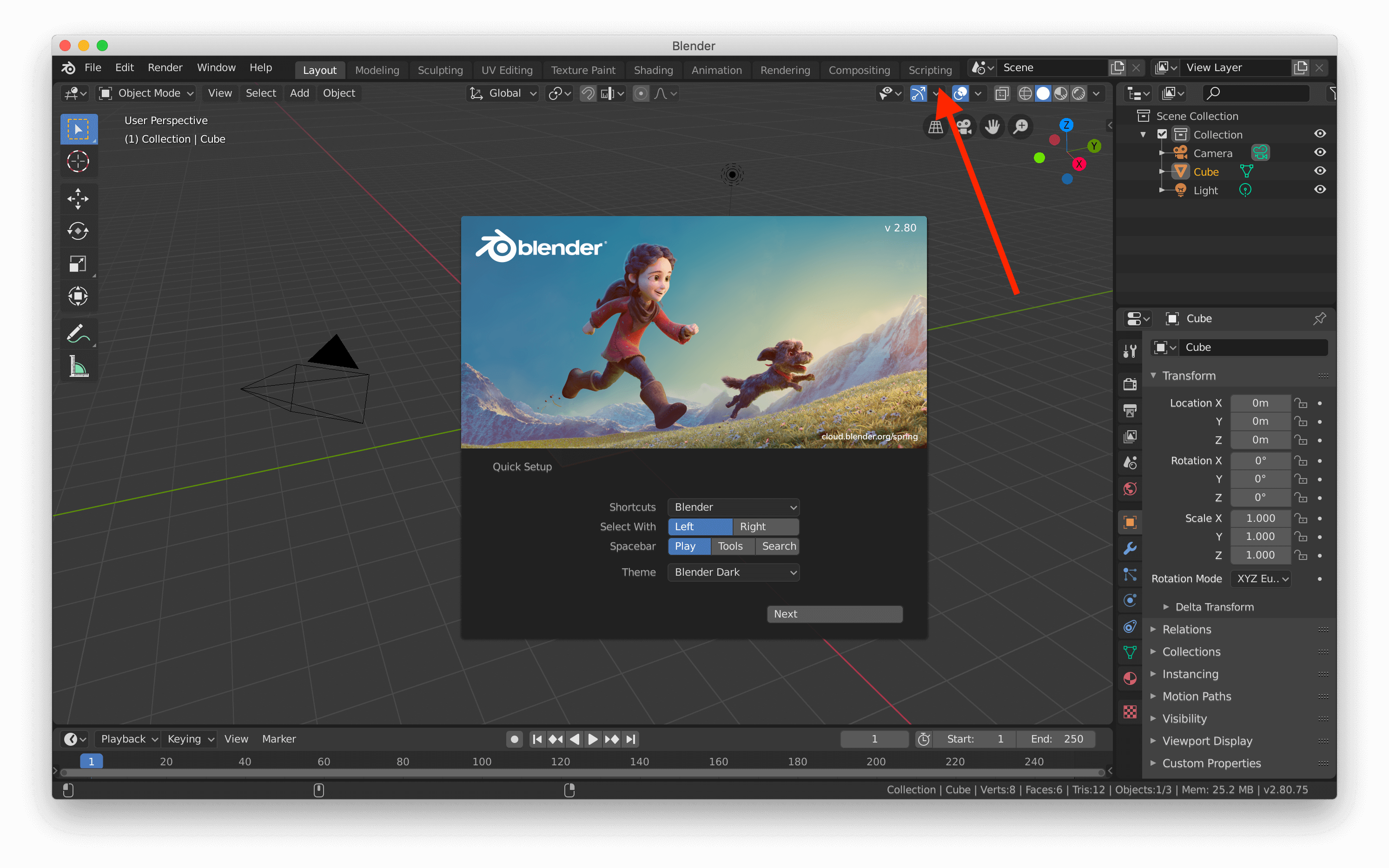
There is a Console
in Scripting
, which works out of the box, and you can enter Python code directly into it.
A century of tradition, let’s hello world:
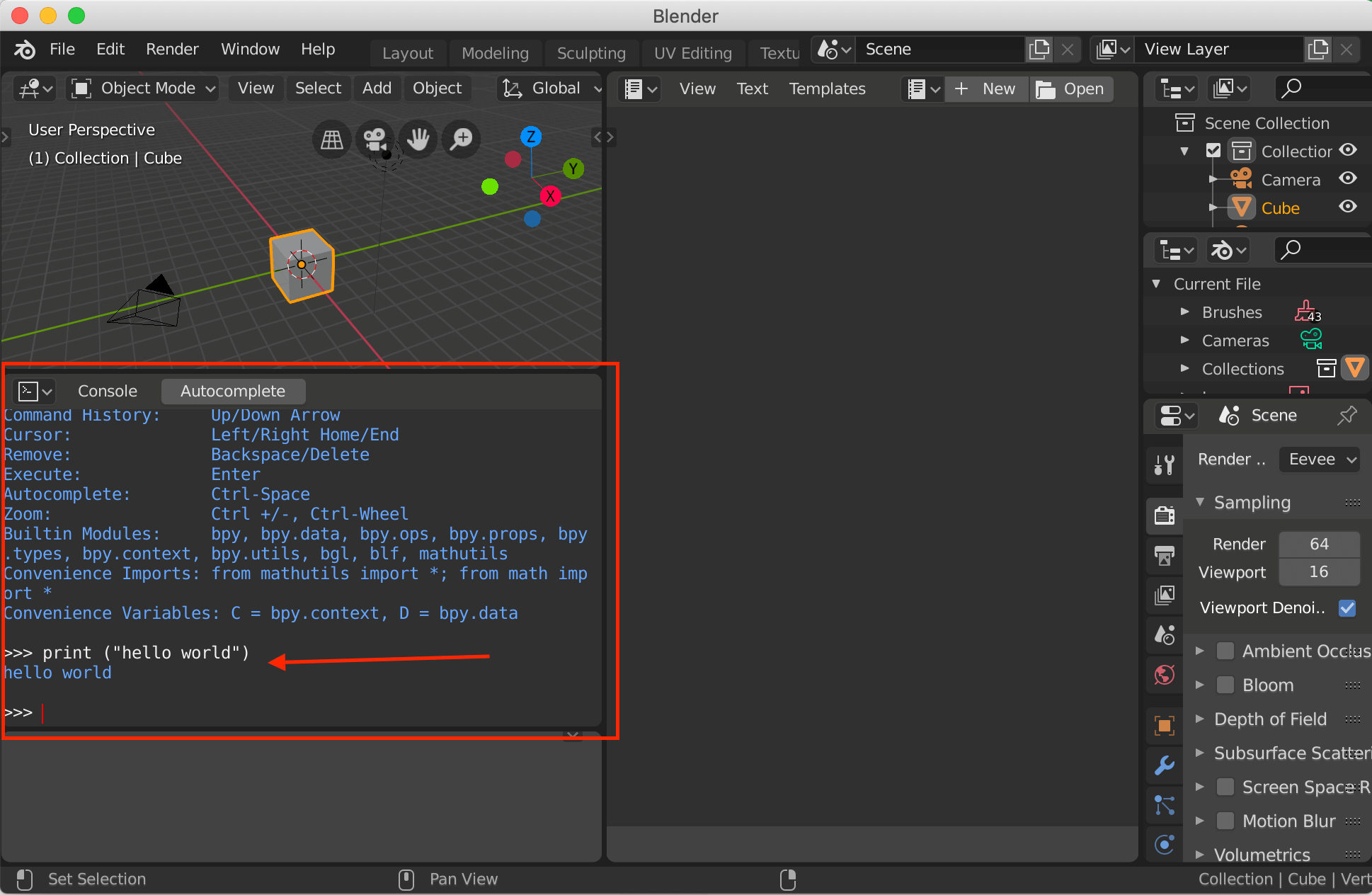
It is inconvenient to write only in the Console
. So click Text
in the picture. This is actually a code text editor. Click New
to start playing:
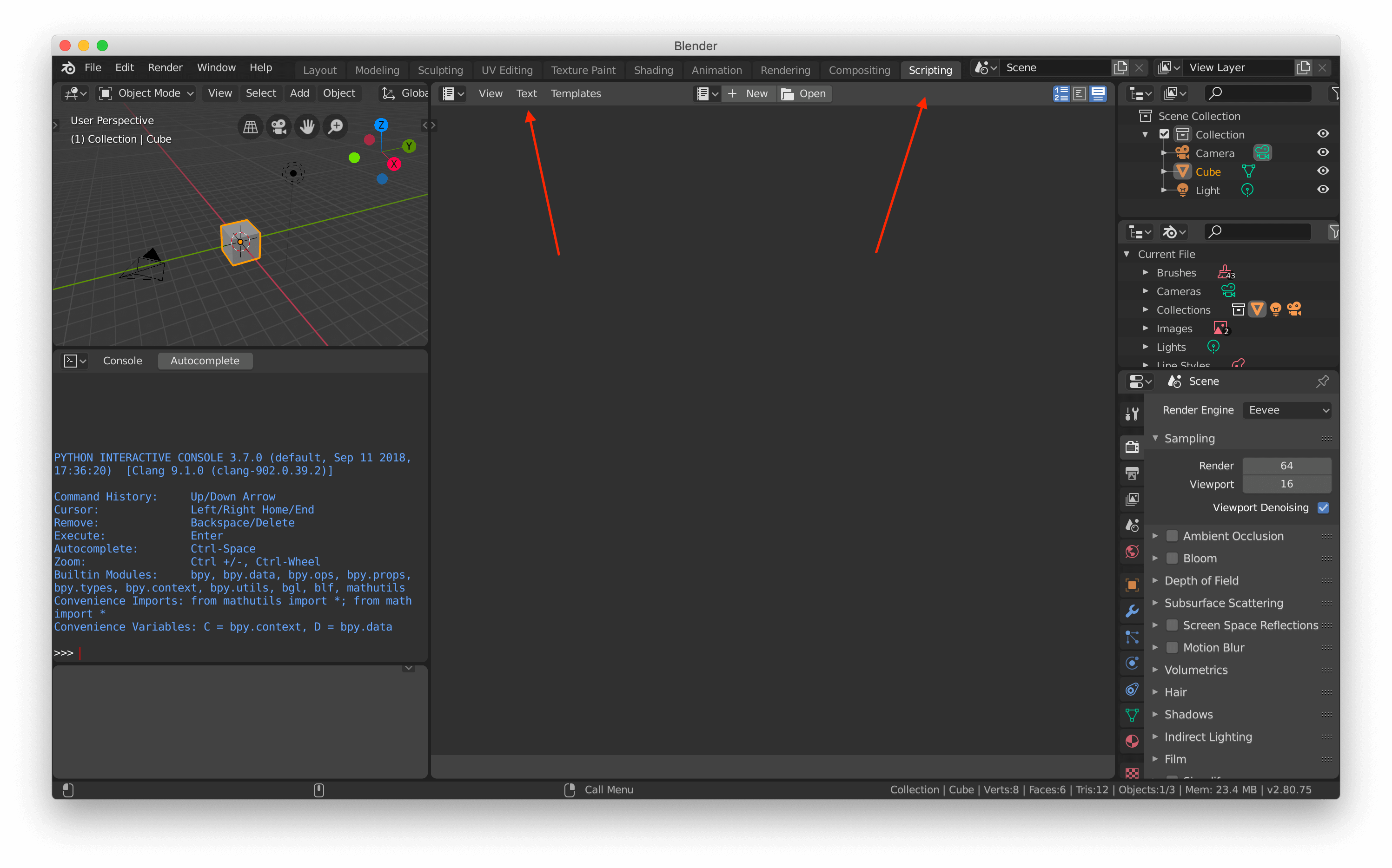
Try hello world agian:
print ("hello world from Text Editor")
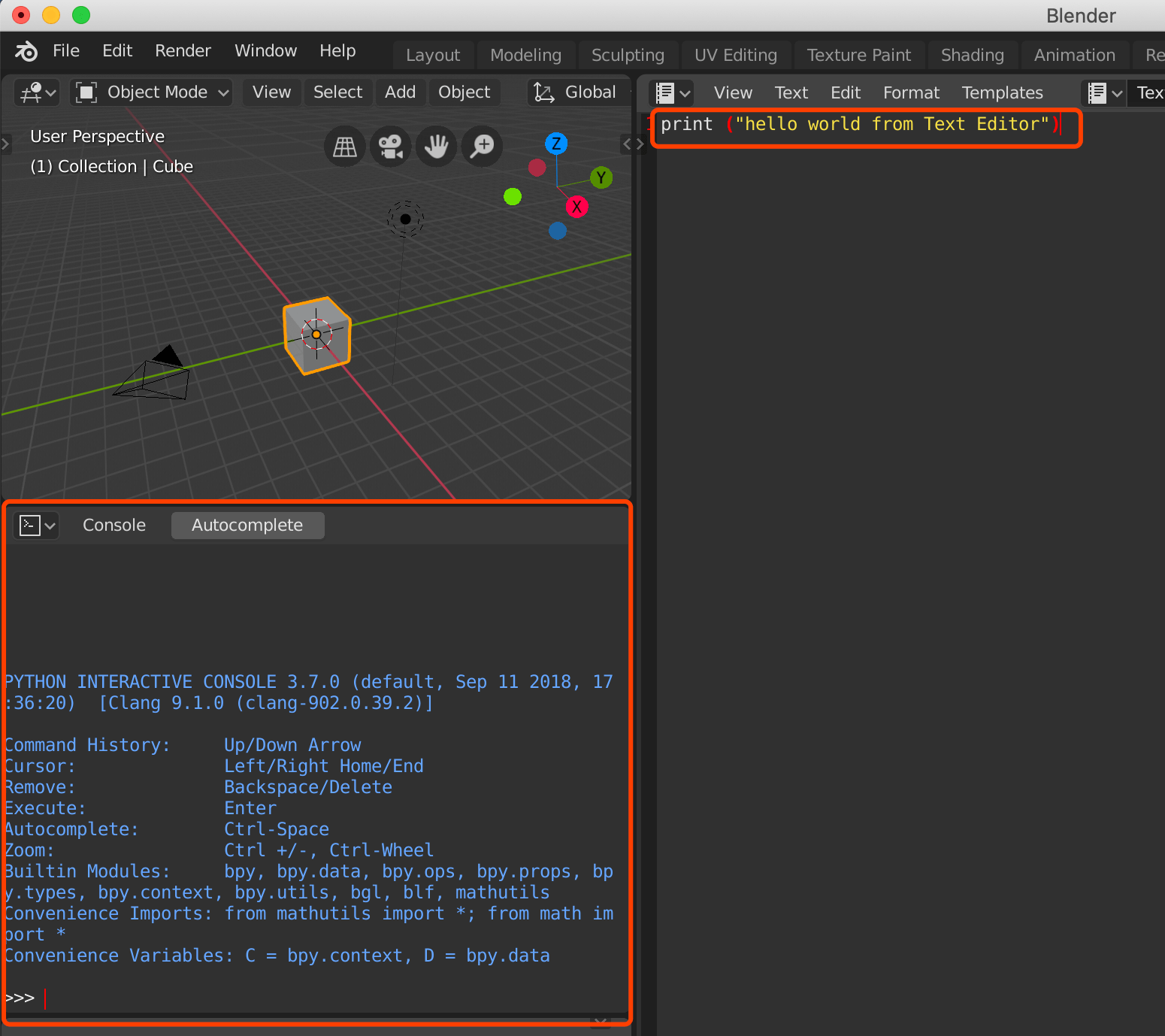
Input code and click Run Script
. You can see that the text “hello world from Text Editor” is not printed in the console area on the left.
To debug the code in Text Editor
you need to open the Console
that comes with the operating system. Offical tips here:
https://docs.blender.org/api/current/info_tips_and_tricks.html#use-the-terminal
For Windows, just open the system Console
in the help
menu.
For Mac, firstly open the system Terminal
(if you have Blender open, close it firstly), then open Blender in Terminal
:
- Open
Terminal
- Right click on Blender (.app) in the
Application
and select “Show Package Contents” or “View Package Contents” - Find Contents-> MacOS-> Blender
- Drag
Blender
into theTerminal
window - Enter
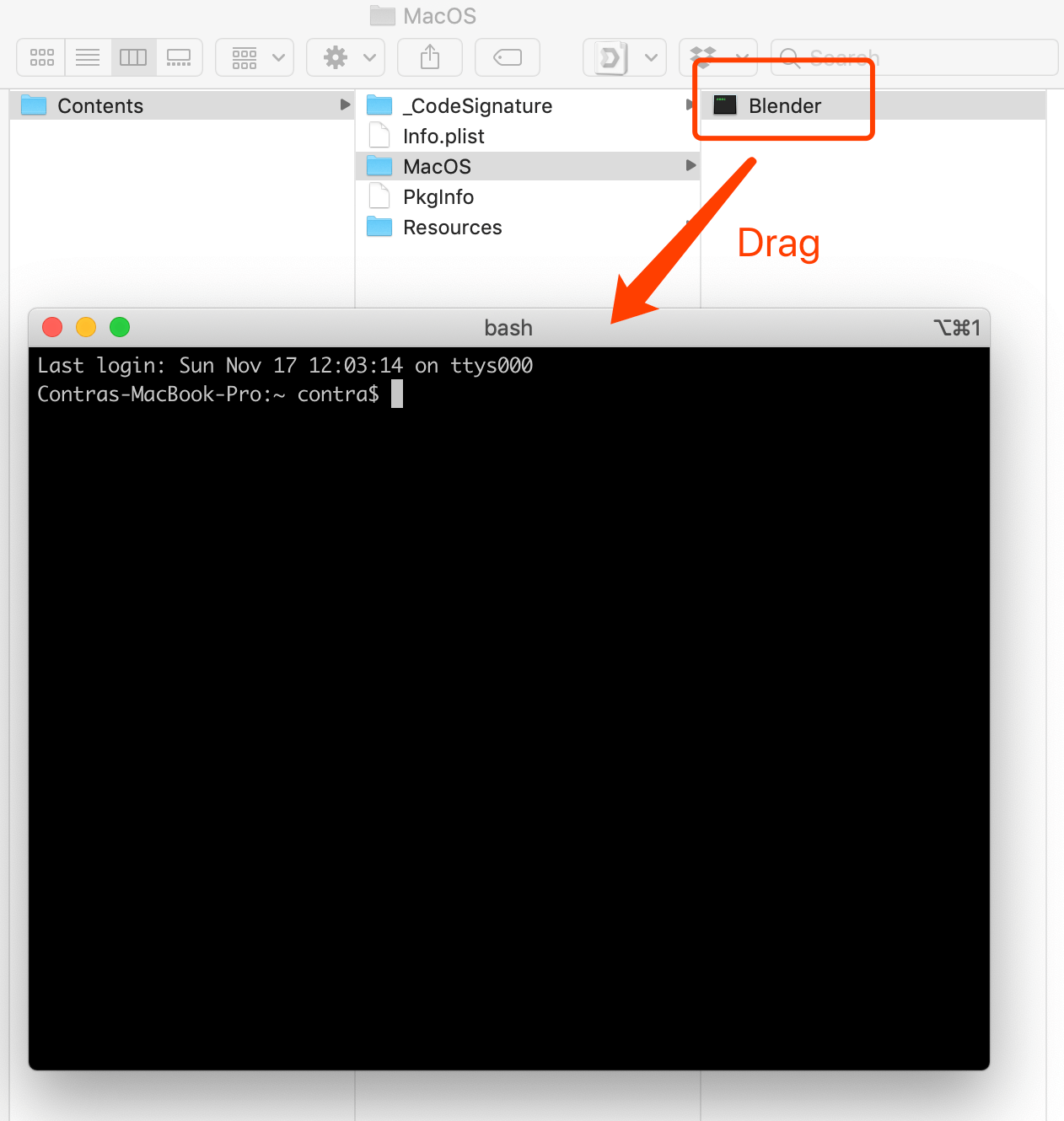
After opening the system Terminal
, re-enter the text in Blender Scripting
:print ("hello world from Text Editor")
Then Run Script
, and now you can find the info printed in Terminal
:
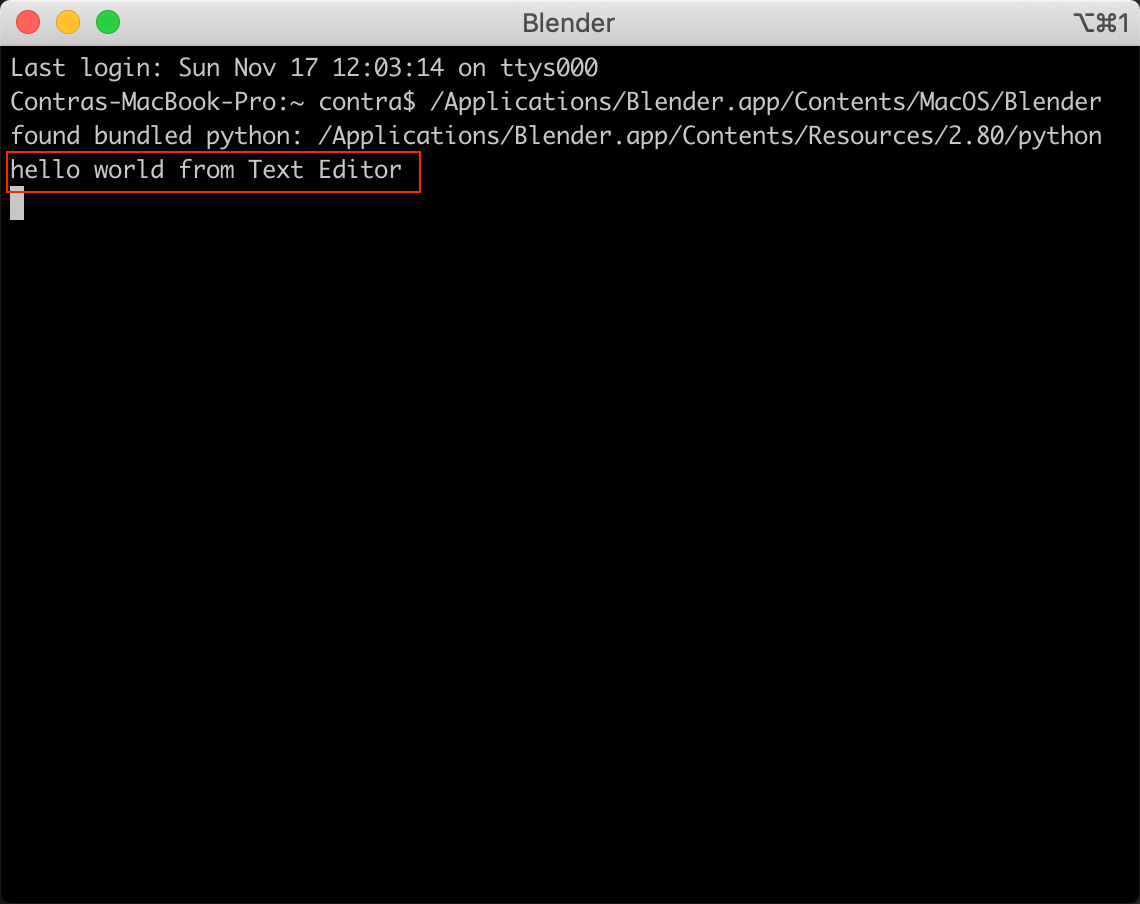
Grease Pencil
Grease Pencil is a new feature of Blender version 2.80. It is a special Blender object that allows you to draw 2D graphics in 3D space, make traditional 2D animations, cut, move, and even make storyboards.
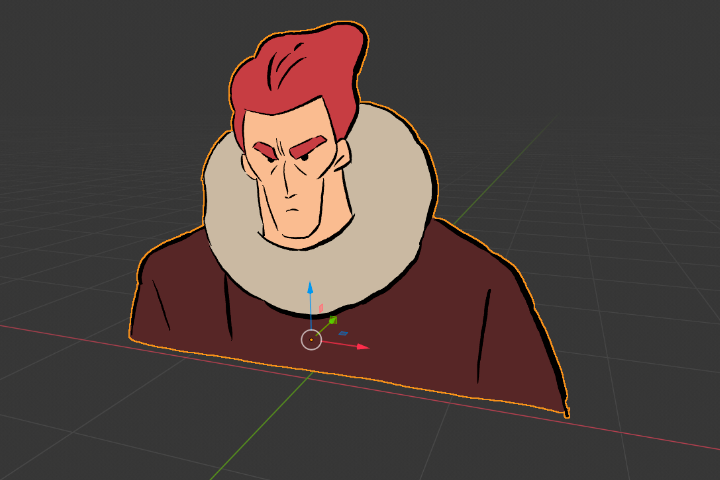
An example of a Grease Pencil object in the 3D environment.
As mentioned earlier, Blender is a software deeply integrated with Python. Basically all software operations have corresponding Python APIs to use.
After laying so much ground, it can finally be summarized as:
Drawing 2D graphics in Blender with calling Grease Pencil API using Python.
It’s a bit like drawing with code in Processing and P5js, but at the same time, you can use Blender’s powerful software functions directly to modify the textures, materials, etc.
At this point, it’s a bit like Unity’s operation.
If unfold Grease Pencil, this article will go further and further. So just list the reference resources here:
- Grease Pencil offical doc:
https://docs.blender.org/manual/en/latest/grease_pencil/introduction.html - Blender Python offical doc:
https://docs.blender.org/manual/en/dev/advanced/command_line/index.html
https://docs.blender.org/manual/en/dev/advanced/scripting/introduction.html - Blender & Grease Pencil API offical doc:
https://docs.blender.org/api/current/ - Blender 2.8 Grease Pencil Scripting and Generative Art
https://towardsdatascience.com/blender-2-8-grease-pencil-scripting-and-generative-art-cbbfd3967590
Note the bold content above: Blender 2.8 Grease Pencil Scripting and Generative Art, its author has encapsulated the commonly used drawing functions. An excerpt is given below. For details, please click the original text above.
For example, wrap a draw_line
function to draw a line between two points:
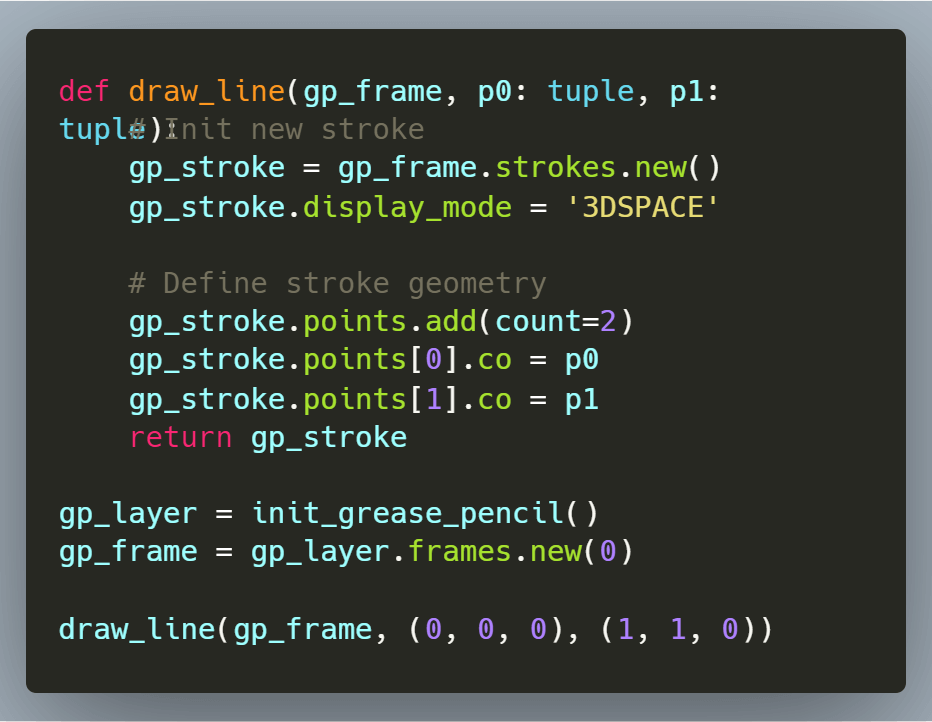
Then draw circles, curves:
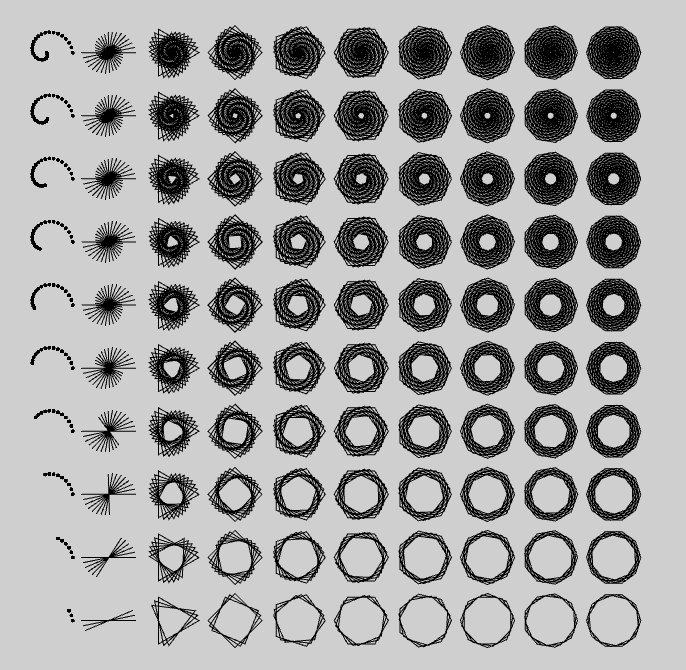
Then use the Material
function of Blender:
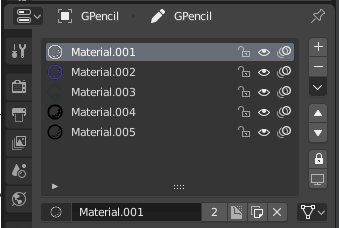
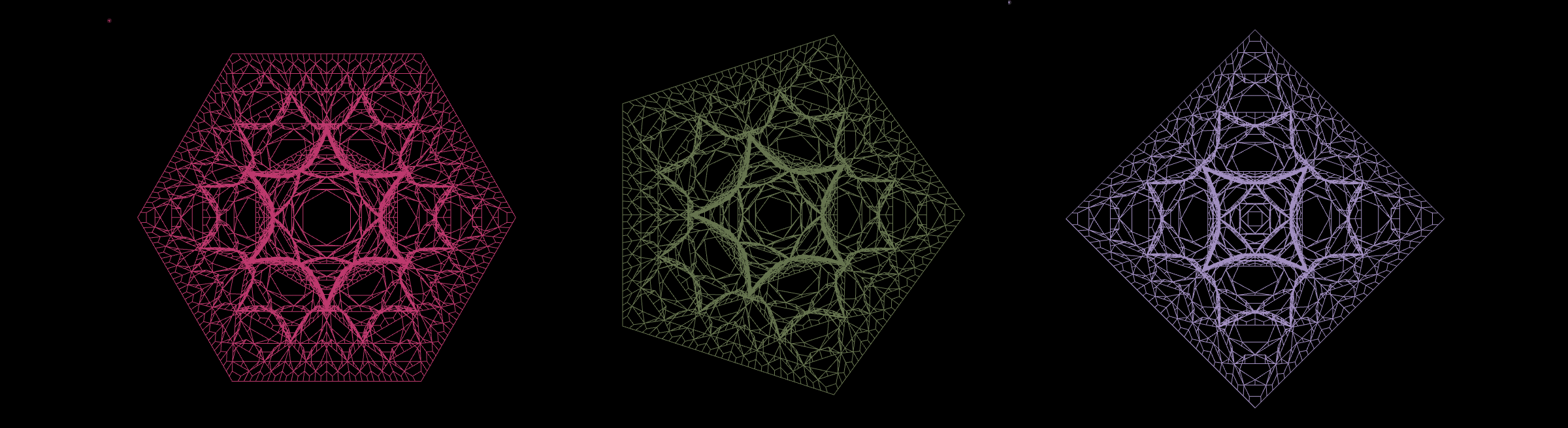
You can even combine Blender’s animation feature:
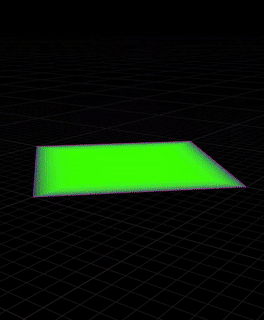
Fourier Series
Finally back to the topic …
Recall the simplified formula of Fourier Series:
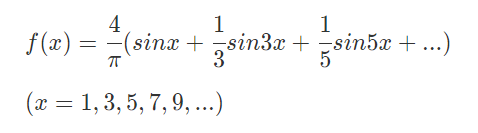
Mathematical knowledge notes on Fourier Series, see Fourier Series Visualization Using React Hooks.
Write formula logic in Python, and call the Blender Grease Pencil API for drawing and rendering:
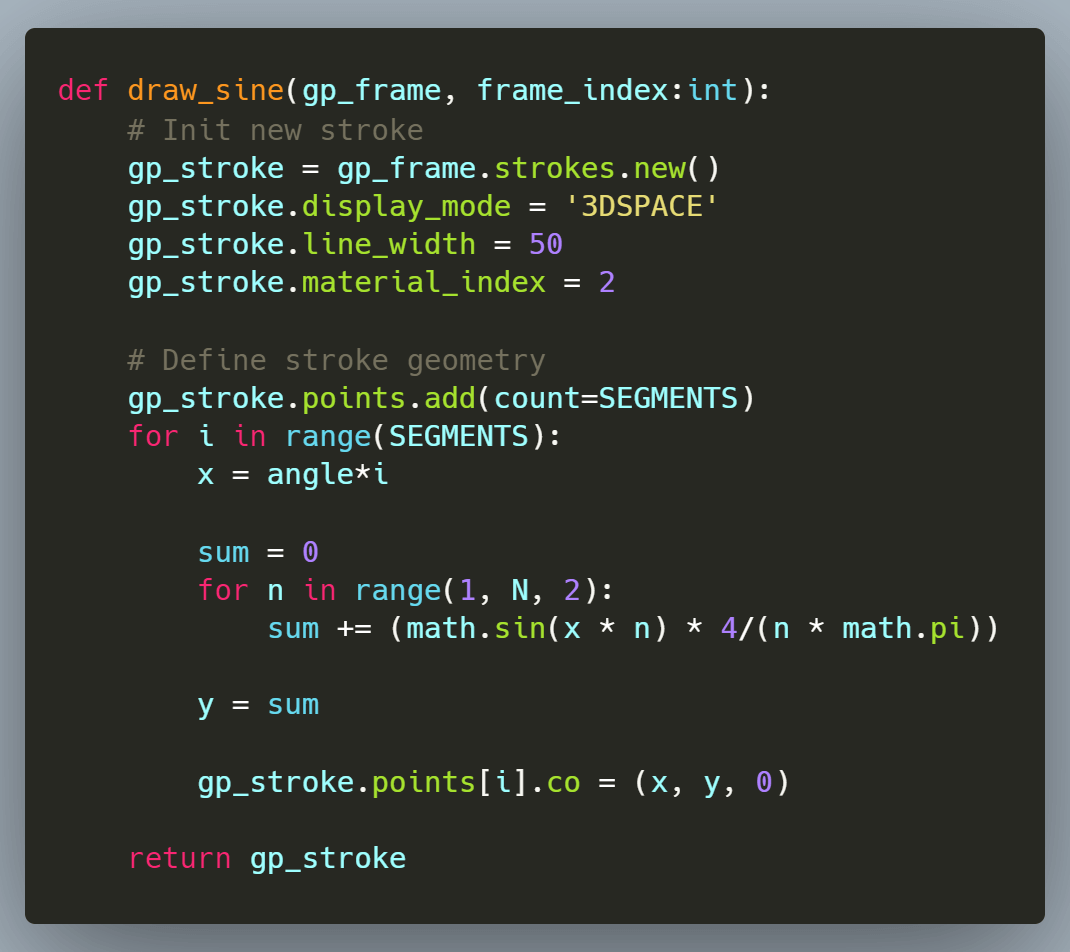
The complete source code can be found later.
Final effect:
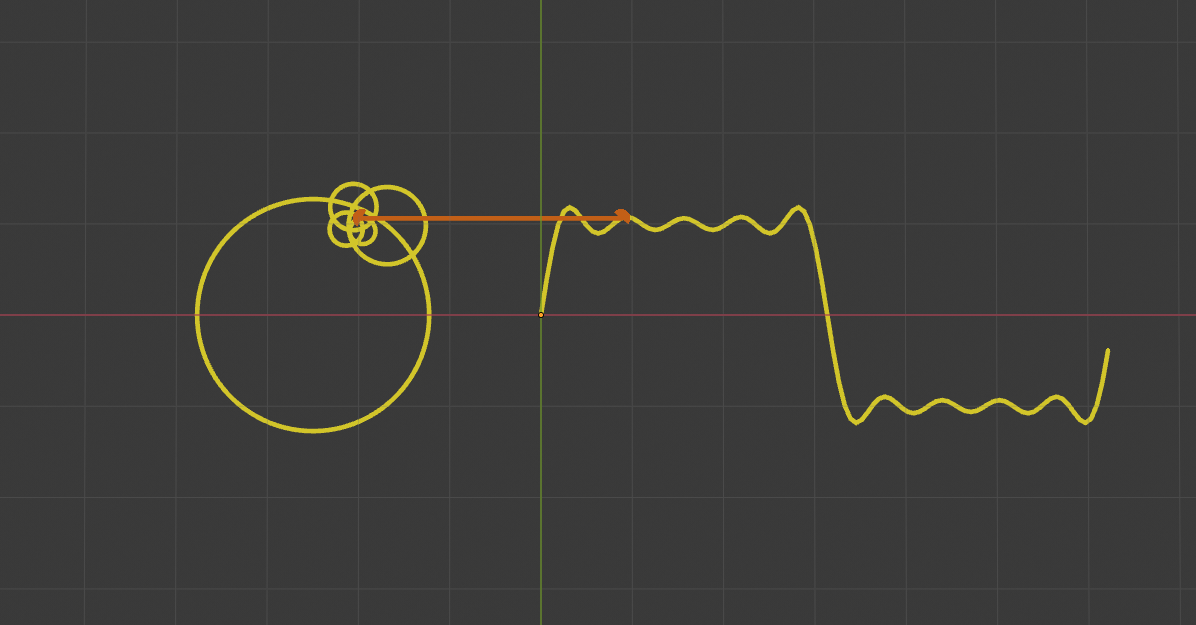
Remember that? Grease Pencil actually draws 2D graphics in 3D space:
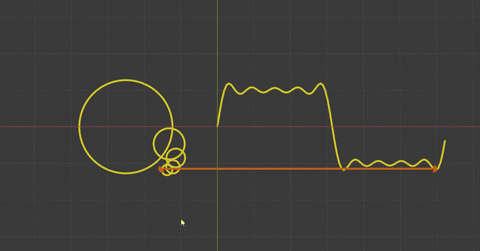
References
- Wikipedia
- Purrier Series (Meow) and Making Images Speak
- CodingChallenges by Daniel Shiffman
- Advanced Mathematics – (Engineering Course) 高等数学(工科类)
- Signals & Systems (Second Edition) by Alan V. Oppenheim
- [干货]—Fourier级数
- 傅立叶变换如何理解?美颜和变声都是什么原理?李永乐老师告诉你
Talk is cheap. Show me the code!
This demo and the Coding Druid series is open source here:
https://github.com/avantcontra/coding-druid
You can find more resources in my site floatbug.com.
You may buy me a coffee in my Patreon. There are many articles, patches, source code and some advanced Patron-only content there.
Also you can get some free patches/codes in Gumroad shop.
Your encouragement is my driving energy!
Cheers~
Contra
- Website: floatbug.com
- Github: avantcontra
- Facebook: avantcontra
- Twitter: avantcontra
- Instagram: avantcontra
- Gumroad (buy codes directly): avantcontra
- Patreon (advanced content): avantcontra
Comments
4 responses to “Using Blender to run Python and visualizing the Fourier Series”
[…] is Python (Blender) version. There are JavaScript (React) version, […]
Thank you very much. I found your blog while looking for a way to draw math graphs in Blender. It helped me so much. I’m very grateful. Have a good day and be healthy.
Cool! My pleasure it could help you a little.
[…] In [Experimental Programming], I generally use Blender as a Python runtime environment out of the box, like:Using Blender to run Python and visualizing the Fourier Series. […]